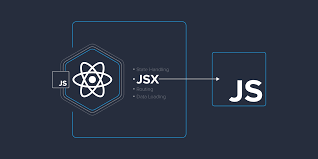
Introduction to JSX
JSX (JavaScript XML) is a syntax extension for JavaScript commonly used with React. It allows developers to write HTML-like code inside JavaScript, making UI development more intuitive. JSX ultimately compiles down to JavaScript function calls, making it both powerful and flexible.
In this blog post, we will cover the fundamentals of JSX, including embedding expressions, using curly braces, and JSX attributes with their differences from standard HTML.
Embedding Expressions in JSX
JSX allows embedding JavaScript expressions directly within the markup. This makes it easy to dynamically render data in React components.
Example:
const name = "John Doe"; const element = <h1>Hello, {name}!</h1>; ReactDOM.render(element, document.getElementById('root'));
In this example, the {name}
expression is evaluated and inserted into the JSX output.
Using Curly Braces {}
in JSX
Curly braces {}
in JSX are used to evaluate JavaScript expressions inside the markup. These expressions can include variables, functions, operations, and even function calls.
Examples:
1. Using Variables:
const age = 25; const element = <p>Age: {age}</p>;
2. Using Functions:
function formatDate(date) { return date.toLocaleDateString(); } const element = <p>Today's Date: {formatDate(new Date())}</p>;
3. Using Conditional (Ternary) Operators:
const isLoggedIn = true; const element = <p>{isLoggedIn ? "Welcome back!" : "Please sign in."}</p>;
JSX Attributes and Differences from HTML
JSX attributes look similar to HTML but with some key differences. Here are some of the main differences:
1. className
Instead of class
In JSX, class
is replaced with className
because class
is a reserved keyword in JavaScript.
const element = <div className="container">Hello, JSX!</div>;
2. camelCase
for Attribute Names
JSX uses camelCase for attribute names, unlike HTML where attributes are usually lowercase.
const element = <button onClick={handleClick}>Click Me</button>;
3. Self-Closing Tags
JSX requires self-closing tags for elements that do not have children.
const image = <img src="image.jpg" alt="A description" />;
4. Boolean Attributes
In JSX, boolean attributes are specified as {true}
or simply their name without a value.
const input = <input type="checkbox" checked={true} />;
Or simply:
const input = <input type="checkbox" checked />;
Conclusion
JSX is a powerful feature of React that makes UI development intuitive and expressive. By understanding JSX expressions, curly braces, and attribute differences from HTML, you can write more efficient and readable React code.
By mastering JSX syntax, you'll be well on your way to building dynamic and interactive user interfaces in React!
Leave a Comment